MySQL CREATE DATABASE Statement
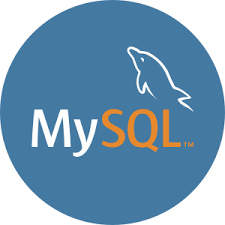
The CREATE DATABASE statement is used to create a new SQL database.
Syntax
CREATE DATABASE databasename;
CREATE DATABASE Example
The following SQL statement creates a database called “testDB”:
Example
CREATE DATABASE testDB;
MySQL DROP DATABASE Statement
The DROP DATABASE statement is used to drop an existing SQL database.
Syntax
DROP DATABASE databasename;
Note: Be careful before dropping a database. Deleting a database will result in loss of complete information stored in the database
DROP DATABASE Example
The following SQL statement drops the existing database “testDB”:
Example
DROP DATABASE testDB;
Tip: Make sure you have admin privilege before dropping any database. Once a database is dropped, you can check it in the list of databases with the following SQL command: SHOW DATABASES;
MySQL Workbench
It is a visual database designing or GUI tool used to work with database architects, developers, and Database Administrators. This visual tool supports SQL development, data modeling, data migration, and comprehensive administration tools for server configuration, user administration, backup, and many more. It allows us to create new physical data models, E-R diagrams, and SQL development (run queries, etc.).
To create a new database using this tool, we first need to launch theMYSQL WORKBENCH and log in using the username and password that you want. It will show the following screen:
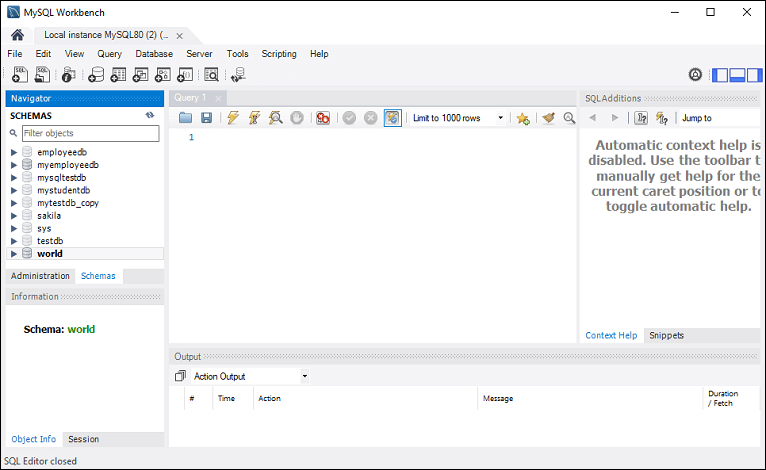
Now do the following steps for database creation:
1. Go to the Navigation tab and click on the Schema menu. Here, we can see all the previously created databases. If we want to create a new database, right-click under the Schema menu and select Create Schema or click the database icon (red rectangle), as shown in the following screen.
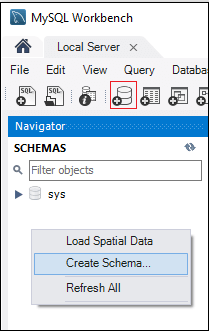
2. The new Schema window screen open. Enter the new database name (for example, employeedb) and use default character set and collation. Now, click on the Apply button as shown in the screen below:
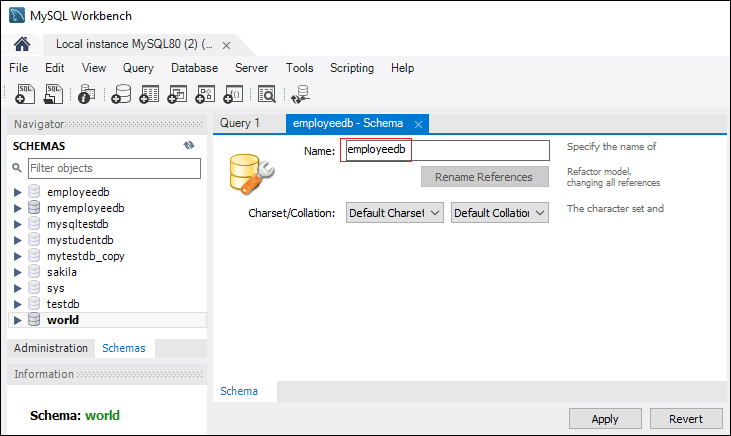
3. A new popup window appears. Click on the Apply button.
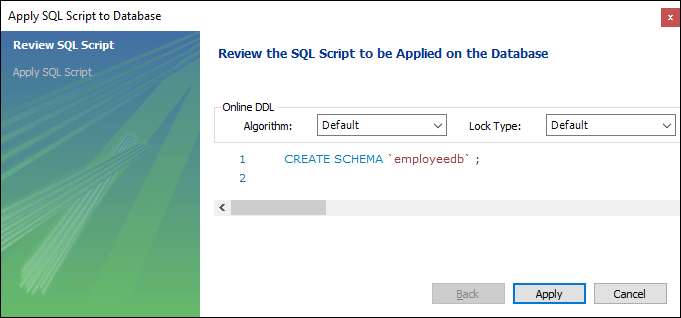
4. A new popup screen appears. Click on the Finish button to complete the database creation.
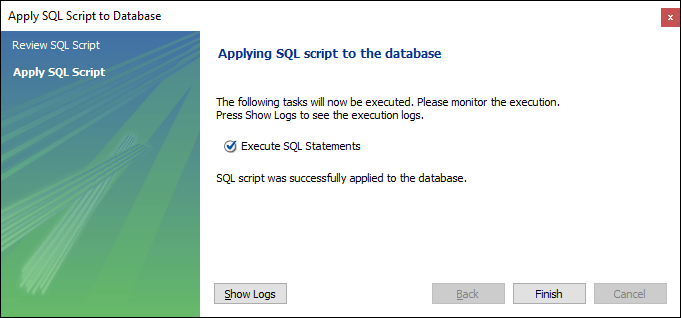
5. After successful database creation, we can see new databases in the Schema menu. If we do not see this, click on the refresh icon into the Schema menu.
6. We can see more information about the database by selecting the database and click on the ‘i’ icon. The information window displays several options, like Table, Triggers, Indexes, Users, and many more.
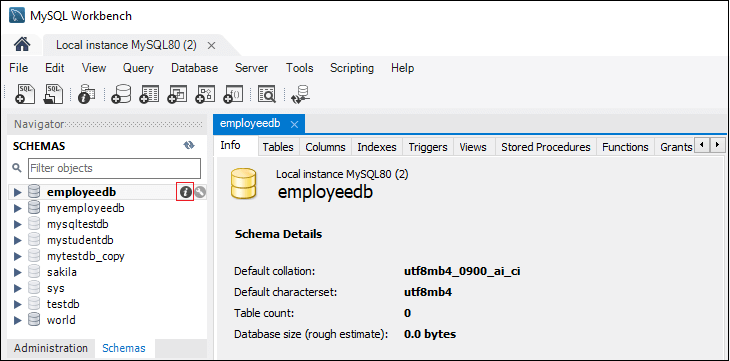
7. MySQL Workbench does not provide an option to rename the database name, but we can create, update, and delete the table and data rows from the database.