#PYTHON(DAY13)
Conditional statements
Python’s conditional statements carry out various calculations or operations according to whether a particular Boolean condition is evaluated as true or false.
Indentation:
Python relies on indentation (whitespace at the beginning of a line) to define scope in the code. Other programming languages often use curly-brackets for this purpose.
If statement, without indentation (will raise an error):
a = 33
b = 200
if b > a:
print("b is greater than a")
And the output is:
File "demo_if_error.py", line 4
print("b is greater than a")
^
IndentationError: expected an indented block
if statement
- ‘if’ keyword is used to validate the conditional expression(c.e) and executes the subsequent code block if the c.e turns out to be ‘True’
- syntax of ‘if’
- ‘if’ conditional_expression :
- code_block
flowchart:
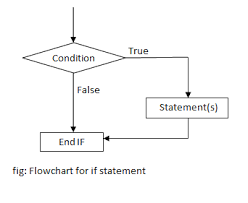
syntax:
if <conditional expression>
Statement
Example:
a, b = 6, 5
# Initializing the if condition
if a > b:
code = "a is greater than b"
print(code)
And the output is:
a is greater than b
if-else:
- if the c.e is true, then the code_block under ‘if’ will be executed
- else the c.e is false, then the code_block under ‘else’ will be executed
- ‘else’ does not have a c.e
flow chart of if-else
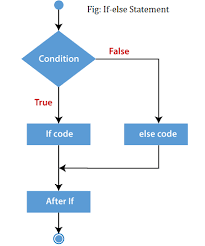
syntax:
if <conditional expression>
Statement
else
Statement
Example:
a = 200
b = 33
if b > a:
print("b is greater than a")
else:
print("b is not greater than a")
And the output is:
a is greater than b
No comments:
Post a Comment