#PYTHON(DAY18)
recurssion function
- recurssion func is a function which calls up on itself
- Note: this kind of fun needs a base case to stop, if not the execution stack will reaches max depth and exhaust the memory( if you are using jupyter notebook the kernel will be dead)
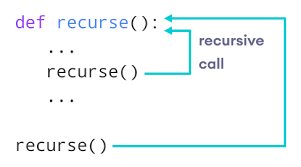
# factorial with using recurssion
def fact(n):
fact = 1
for i in range(1,n+1):
fact = fact*i
n-=1
return fact
fact(4)
And the output is:
24
lambda functions
- these functions are called as anonymous functions
- these are defined in a single line
- syntax for lambda functions:
- ‘lambda’ var_name: <’statement/s using these var/s’>
- these functions by default will return a value, no need to write a return statement
- there is no cap on no of vars one can use to define a lambda function
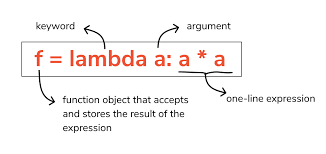
# normal func
def powAB(a,b):
return a**b
powAB(4,5)
And the output is:
1024
filter()
- filter function is used to filter the elements based on user’s logic
- this user’s logic is written as function, which gives a boolean output, True when it meets the user’s logic, or else it will return False
- filter function will return an iterator of cls=ass filter, we can typecast this to a list
- filter function accepts 2 parameters 1) function 2) iterator/container
- syntax of filter function:
- filter(func,container/iterator)
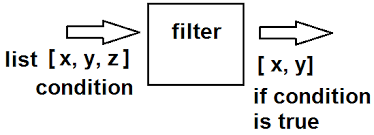
nums = [i for i in range(1,51)]
even = filter(lambda num: num%2 == 0,nums)
odd = filter(lambda num: num%2 !=0, nums)
print(type(even),type(odd))
even,odd = list(even),list(odd)
print(type(even),type(odd))
print(even,odd,sep = '\n')
And the output is:
<class 'filter'> <class 'filter'>
<class 'list'> <class 'list'>
[2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24, 26, 28, 30, 32, 34, 36, 38, 40, 42, 44, 46, 48, 50]
[1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29, 31, 33, 35, 37, 39, 41, 43, 45, 47, 49]
map
- we use ‘map’ function to apply our function on each element of an iterator
- syntax of ‘map’ function:
- map(func,iterator)
- output of map function is a map object, we need to type cast it to a list or other data types to see the output
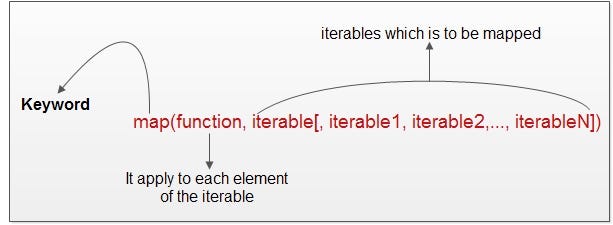
nums = [ele for ele in range(1,11)]
cube_nums = map(lambda x : x**3, nums)
print(cube_nums, type(cube_nums), sep = '\n')
cube_nums = list(cube_nums)
print(cube_nums, type(cube_nums),sep = '\n')
And the output is:
<map object at 0x00000230D2B6B700>
<class 'map'>
[1, 8, 27, 64, 125, 216, 343, 512, 729, 1000]
<class 'list'>
No comments:
Post a Comment