#PYTHON(DAY6)
List and list indexing in python
Lists
- lists are a collection in python, and they are heterogeneous in nature
- means they can store multiple elements which belong to multiple data types/classes
- we seperate elemts in list by ‘,’
- lists are denoted by ‘[]’, we enclose our elements within square braces
- empty list = ‘[]’
- lists are ordered and sequence in nature
- If you add new items to a list, the new items will be placed at the end of the list.
- lists can be indexed and can be sliced
- when calling a method results in updation of list, these updations are reflected in same memory location and are applied on same variable, unlike string, we don not have reassign to make them reflect original var
- list are dynamic in nature
x = [1,2.2,3.3j,'four',print,str,True]
print(x,len(x),type(x))
And the expected output for the above code is [1, 2.2, 3.3j, ‘four’, <built-in function print>, <class ‘str’>, True] 7 <class ‘list’>
Since lists are indexed, lists can have items with the same value:
print(x[0], x[-1], sep ='\n')
INDEX()
- index will return the index position of first occurence of given element
- index method throws an error if a value/element is passed which is not in their list
x = [1,2,3,4]
x.index(5)
we get the value error
INDEXING
Positive Indexing
Indexing in Python starts at 0, which means that the first element in a sequence has an index of 0, the second element has an index of 1, and so on. For example, if we have a string “Hello”, we can access the first letter “H” using its index 0 by using the square bracket notation: string[0] .
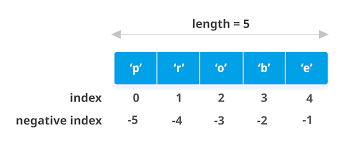
List items are indexed and you can access them by referring to the index number:
thislist = ["apple", "banana", "cherry"]
print(thislist[1])
Negative Indexing
Negative indexing means start from the end
-1 refers to the last item, -2 refers to the second last item etc.
thislist = ["apple", "banana", "cherry"]
print(thislist[-1])
range
- range() populates a numbers within a given range.
- syntax for range()range(start,end,step)
- start is starting number
- end is till end, not encluding and
- step is the step size
- like in strings, we have a negative step in range
- default values of start is zero and step is +1
You can specify a range of indexes by specifying where to start and where to end the range.
When specifying a range, the return value will be a new list with the specified items.
thislist = ["apple", "banana", "cherry", "orange", "kiwi", "melon", "mango"]
print(thislist[2:5])
Note: The search will start at index 2 (included) and end at index 5 (not included).
x = range(1,21,3)
y = range(20,0,-2)
#z = range(20.5,1.5,0.5) # can not access float numbers
print(x, id(x))
print(y, id(y))
And the output is: range(1, 21, 3) 2590467408064
range(20, 0, -2) 2590467408832
x = list(x)
print(x, type(x))
print()
y = list(y)
print(y,type(y))
And the output is: [1, 4, 7, 10, 13, 16, 19] <class ‘list’>
[20, 18, 16, 14, 12, 10, 8, 6, 4, 2] <class ‘list’>
x = range(3, 20, 2)
for n in x:
print(n)
Expected output is : 3
5
7
9
11
13
15
17
19
No comments:
Post a Comment