MySQL GROUP BY Statement
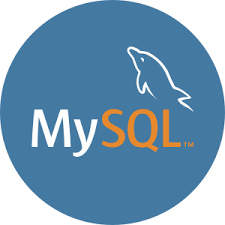
The GROUP BY statement groups rows that have the same values into summary rows, like "find the number of customers in each country".
The GROUP BY statement is often used with aggregate functions (COUNT() , MAX() , MIN() , SUM() , AVG() ) to group the result-set by one or more columns.
GROUP BY Syntax
SELECT column_name(s)
FROM table_name
WHERE condition
GROUP BY column_name(s)
ORDER BY column_name(s);
Demo Database
Below is a selection from the “Customers” table in the sample database:
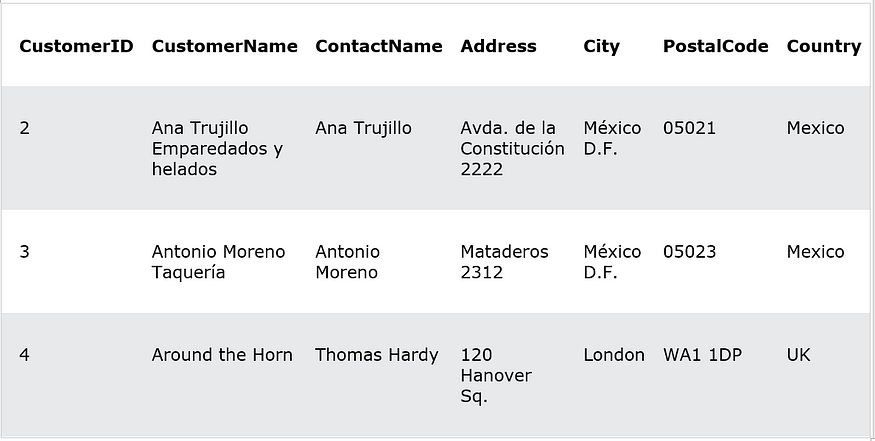
MySQL GROUP BY Examples
The following SQL statement lists the number of customers in each city:
Example
SELECT COUNT(CustomerID), City
FROM Customers
GROUP BY City;
The following SQL statement lists the number of customers in each city, sorted high to low:
Example
SELECT COUNT(CustomerID), City
FROM Customers
GROUP BY City
ORDER BY COUNT(CustomerID) DESC;
Demo Database
Below is a selection from the “Orders” table in the Northwind sample database:

And a selection from the “Shippers” table:

GROUP BY With JOIN Example
The following SQL statement lists the number of orders sent by each shipper:
Example
SELECT Shippers.ShipperName, COUNT(Orders.OrderID) AS NumberOfOrders FROM Orders
LEFT JOIN Shippers ON Orders.ShipperID = Shippers.ShipperID
GROUP BY ShipperName;