MySQL UPDATE Statement
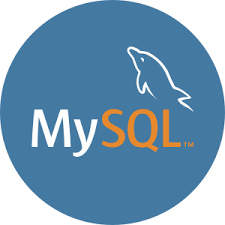
The UPDATE statement is used to modify the existing records in a table.
UPDATE Syntax
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
Note: Be careful when updating records in a table! Notice the WHERE clause in the UPDATE statement. The WHERE clause specifies which record(s) that should be updated. If you omit the WHERE clause, all records in the table will be updated!
Demo Database
Below is a selection from the “Employees” table in the sample database:
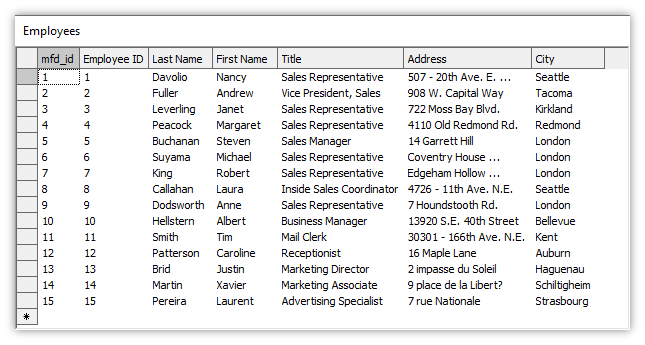
UPDATE Table
The following SQL statement updates the first Employees(EmployeeID = 1) with a new contact person and a new city.
Example
UPDATE Employees
SET LastName = 'Davolio', City = 'Seattle'
WHERE EmployeeID = 1;
UPDATE Multiple Records
It is the WHERE clause that determines how many records will be updated.
The following SQL statement will update the City to “London” for all records where City is “Seattle”:
Example
UPDATE Employees
SET City = 'London'
WHERE City = 'Seattle';