Building Static Website(part 3)
Favourite places detailed view section
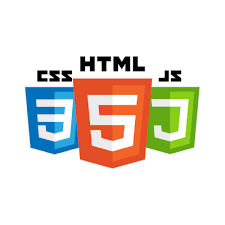
Today let us learn how to add the detailed view sections for our favourite places.
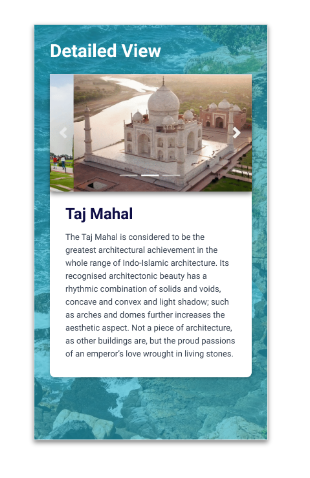
1. Bootstrap Components
Carousel
The carousel is a slideshow where different images or text will change for every few seconds.
To add the Carousel to our Favourite Place Detailed View Section Page, we used Bootstrap Carousel Component with the Indicators.
You can add the different images in the Carousel by changing the image URL in the value of the HTML src attribute.
For Example:
This is how the carousel looks like
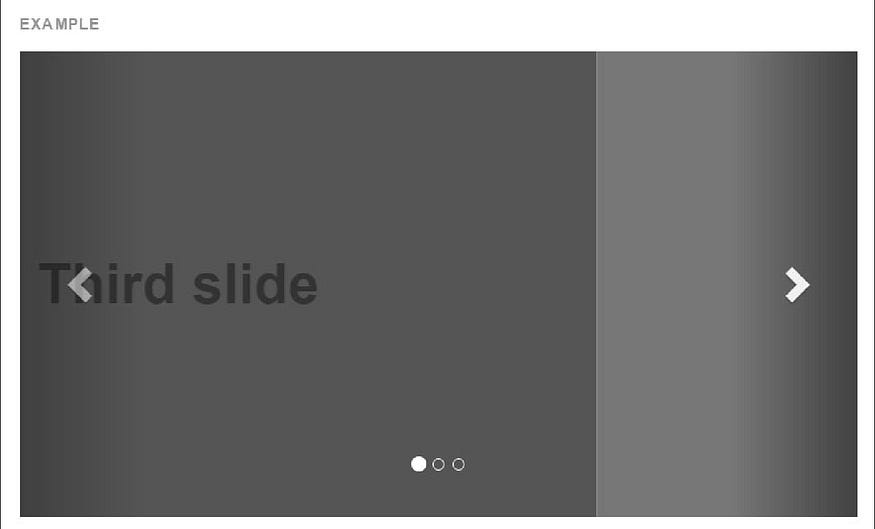
Now let us start building Favourite places detailed section.
Add the background image:
As we have discussed in the previous stories about adding the background image. I f you have missed reading it you can go through here
https://medium.com/@bhavithach8/introduction-to-css-box-model-static-website-eb457051e0e9
<html>
<head>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script>
<link rel="stylesheet" href="favdet.css">
</head>
<body>
<div class="bg-container">
</div>
</body>
</html>
@import url('https://fonts.googleapis.com/css2?family=Bree+Serif&family=Caveat:wght@400;700&family=Lobster&family=Monoton&family=Open+Sans:ital,wght@0,400;0,700;1,400;1,700&family=Playfair+Display+SC:ital,wght@0,400;0,700;1,700&family=Playfair+Display:ital,wght@0,400;0,700;1,700&family=Roboto:ital,wght@0,400;0,700;1,400;1,700&family=Source+Sans+Pro:ital,wght@0,400;0,700;1,700&family=Work+Sans:ital,wght@0,400;0,700;1,700&display=swap');
.bg-container{
background-image:url("images/detaback.jpg");
background-size:cover;
}
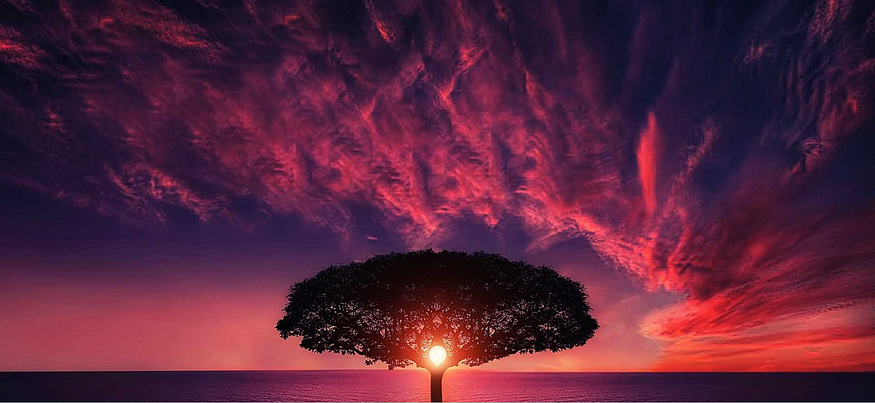
This is how the background image is being added.
Adding Main Heading:
Let us add main heading to the favourite place detailed view. New div section should be added inside the bg-container.
<div>
<h1 class="mainheading">Detailed View</h1>
</div>
.mainheading{
font-family:"Roboto";
font-size:40px;
color:white;
font-weight:bold;
padding:20px;
}
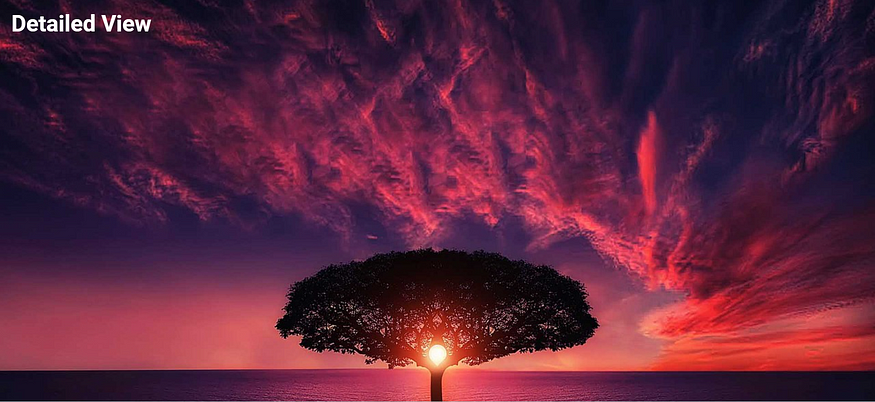
Adding Card Container:
Now let us add the card container.
<div class="container-card">
</div>
.container-card{
background-color:white;
border-bottom-left-radius:8px;
border-bottom-right-radius:8px;
margin:20px;
}
As there is no content in the card container tnere will be no difference in the output.
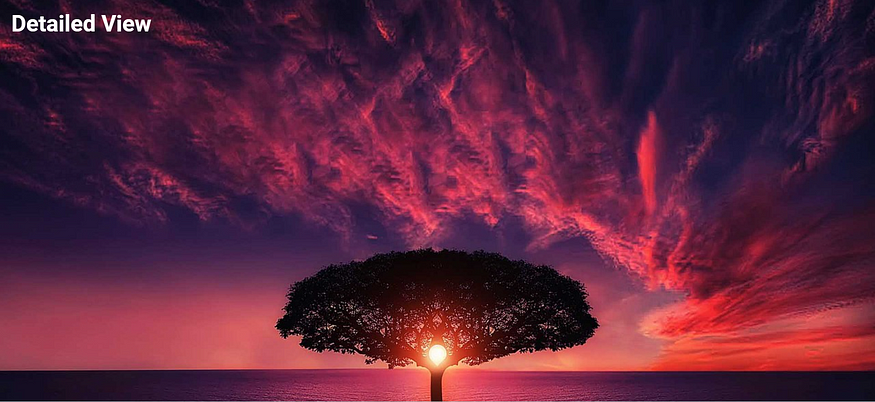
Adding Carousel:
Inorder to add the carousel you need to go to https://getbootstrap.com/ and from there you select documents, in the documents find for the components and you can find carousel over there and it is from the version 4.5.
Now copy the code of carousel with indicators and paste it inside the container card.
place the image url of the images that you choose place in the carousel within the empty img src.
<div id="carouselExampleIndicators" class="carousel slide" data-ride="carousel">
<ol class="carousel-indicators">
<li data-target="#carouselExampleIndicators" data-slide-to="0" class="active"></li>
<li data-target="#carouselExampleIndicators" data-slide-to="1"></li>
<li data-target="#carouselExampleIndicators" data-slide-to="2"></li>
</ol>
<div class="carousel-inner">
<div class="carousel-item active">
<img src="images/tajmahal1.jpg" class="d-block w-100" alt="...">
</div>
<div class="carousel-item">
<img src="images/tajmahal4.jpg" class="d-block w-100" alt="...">
</div>
<div class="carousel-item">
<img src="images/tajmahal5.jpg" class="d-block w-100" alt="...">
</div>
</div>
<a class="carousel-control-prev" href="#carouselExampleIndicators" role="button" data-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="sr-only">Previous</span>
</a>
<a class="carousel-control-next" href="#carouselExampleIndicators" role="button" data-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="sr-only">Next</span>
</a>
</div>
.container-card{
background-color:white;
border-bottom-left-radius:8px;
border-bottom-right-radius:8px;
margin:20px;
}
And as shown below the images within the carousel are added:
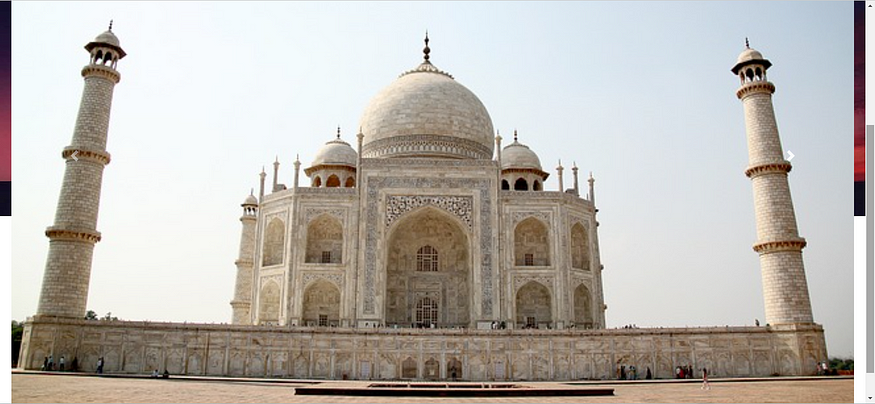
Adding the text container:
Now let us add the text container for the card heading and the description.
<div class="text-container">
<h1 class="heading1">Taj Mahal</h1>
<p class="paragrapg">If there was just one symbol to represent all of India, it would be the Taj Mahal.It is a masterpiece of architectural style in conception, treatment and execution and has unique aesthetic qualities in balance, symmetry and harmonious blending of various elements.</p>
</div>
.text-container{
padding:16px;
}
.heading1{
font-family:"Roboto";
font-size:25px;
font-weight:bold;
margin:10px;
}
.paragraph{
font-size:20px;
font-family:"Roboto";
padding:15px;
}
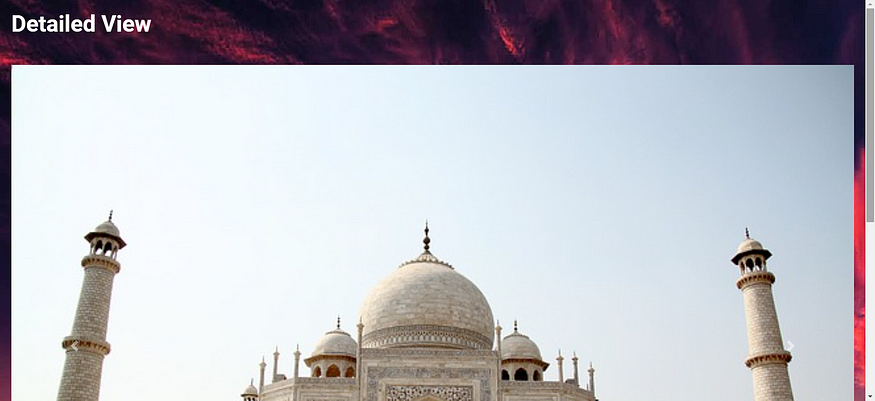
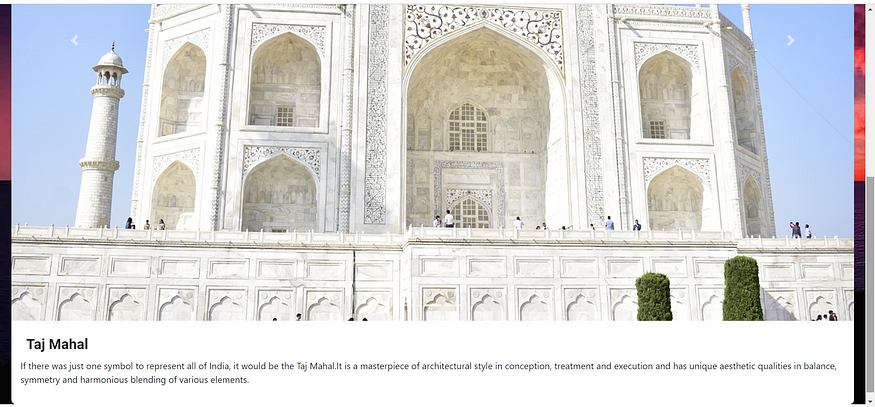
Adding video in the place of carousel:
In the same way let us go to getbootstrap and the go to documents → utlities → below example → copy the two lines of code.
For Example:
<div class="embed-responsive embed-responsive-16by9">
<iframe class="embed-responsive-item" src="https://www.youtube.com/embed/zpOULjyy-n8?rel=0" allowfullscreen></iframe>
</div>
And you need to place the video ID after the embed/ as shown below:
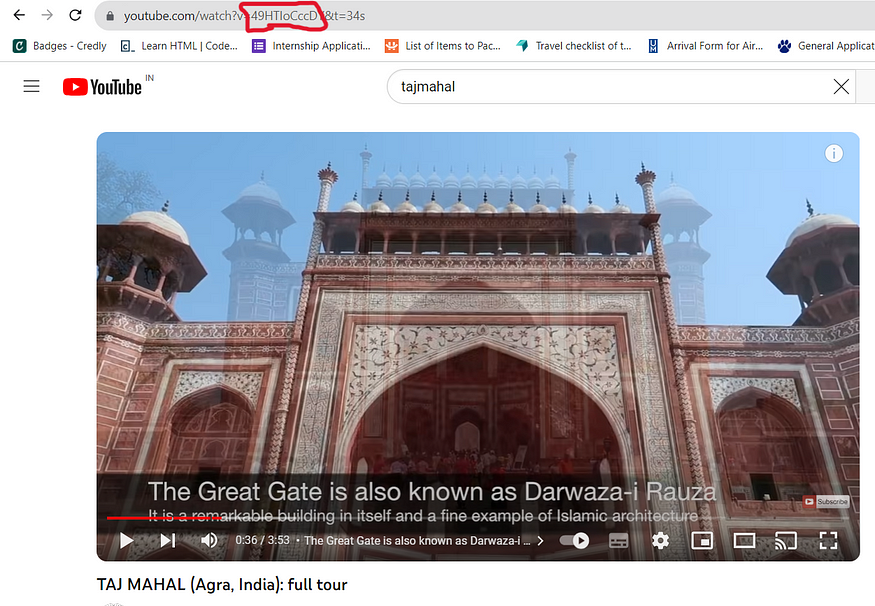
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" integrity="sha384-JcKb8q3iqJ61gNV9KGb8thSsNjpSL0n8PARn9HuZOnIxN0hoP+VmmDGMN5t9UJ0Z" crossorigin="anonymous">
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.1/dist/umd/popper.min.js" integrity="sha384-9/reFTGAW83EW2RDu2S0VKaIzap3H66lZH81PoYlFhbGU+6BZp6G7niu735Sk7lN" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="sha384-B4gt1jrGC7Jh4AgTPSdUtOBvfO8shuf57BaghqFfPlYxofvL8/KUEfYiJOMMV+rV" crossorigin="anonymous"></script>
<link rel="stylesheet" href="favdet.css">
</head>
<body>
<div class="bg-container">
<h1 class="mainheading">Detailed View</h1>
<div class="container-card">
<div class="embed-responsive embed-responsive-16by9">
<iframe class="embed-responsive-item" src="https://www.youtube.com/embed/49HTIoCccDY?rel=0" allowfullscreen></iframe>
</div>
<div class="text-container">
<h1 class="heading1">Taj Mahal</h1>
<p class="paragraph">If there was just one symbol to represent all of India, it would be the Taj Mahal.It is a masterpiece of architectural style in conception, treatment and execution and has unique aesthetic qualities in balance, symmetry and harmonious blending of various elements.</p>
</div>
</div>
</div>
</body>
</html>
@import url('https://fonts.googleapis.com/css2?family=Bree+Serif&family=Caveat:wght@400;700&family=Lobster&family=Monoton&family=Open+Sans:ital,wght@0,400;0,700;1,400;1,700&family=Playfair+Display+SC:ital,wght@0,400;0,700;1,700&family=Playfair+Display:ital,wght@0,400;0,700;1,700&family=Roboto:ital,wght@0,400;0,700;1,400;1,700&family=Source+Sans+Pro:ital,wght@0,400;0,700;1,700&family=Work+Sans:ital,wght@0,400;0,700;1,700&display=swap');
.bg-container{
background-image:url("images/detaback.jpg");
background-size:cover;
}
.mainheading{
font-family:"Roboto";
font-size:40px;
color:white;
font-weight:bold;
padding:20px;
}
.container-card{
background-color:white;
border-bottom-left-radius:8px;
border-bottom-right-radius:8px;
margin:20px;
}
.text-container{
padding:16px;
}
.heading1{
font-family:"Roboto";
font-size:25px;
font-weight:bold;
margin:10px;
}
.paragraph{
font-size:20px;
font-family:"Roboto";
padding:15px;
}
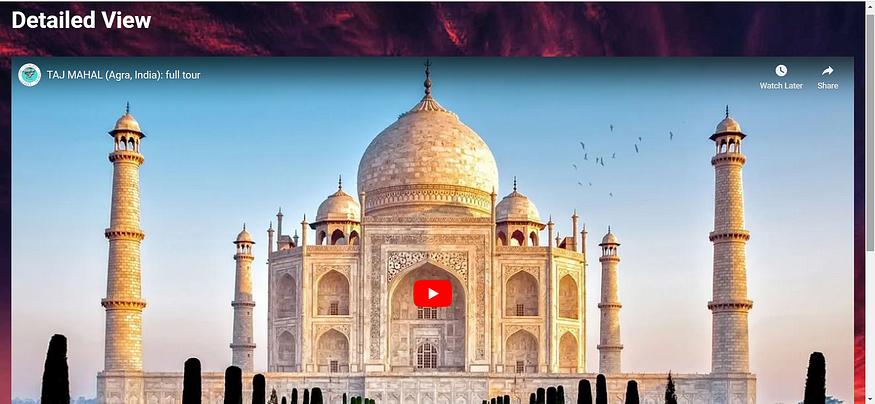
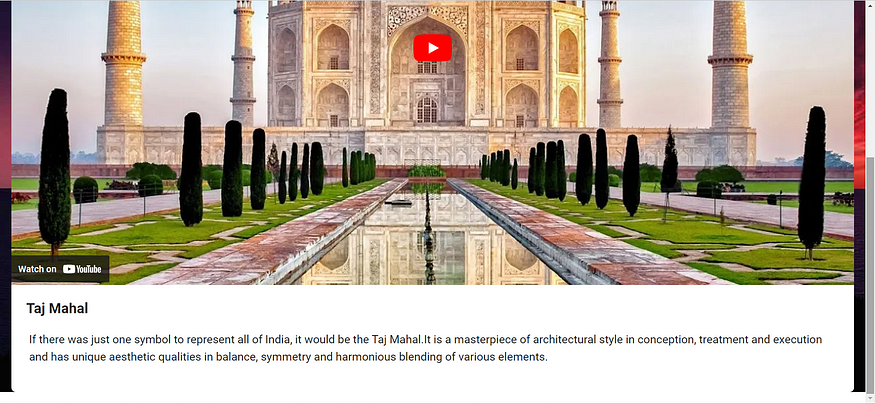
This is how the youtube video is added in the place of carousel.
No comments:
Post a Comment